After going through the HTML and CSS, we started learning about JavaScript. In this post, I wanted to write notes regarding the intro to JavaScript. Some of the topics were a bit confusing to me in the beginning, but everything is getting clear now bit by bit.
Back Story
First, I’d like to mention that I used to learn a coding language when I was 15 or so. It was back in Russia, and the name of the language was Pascal.
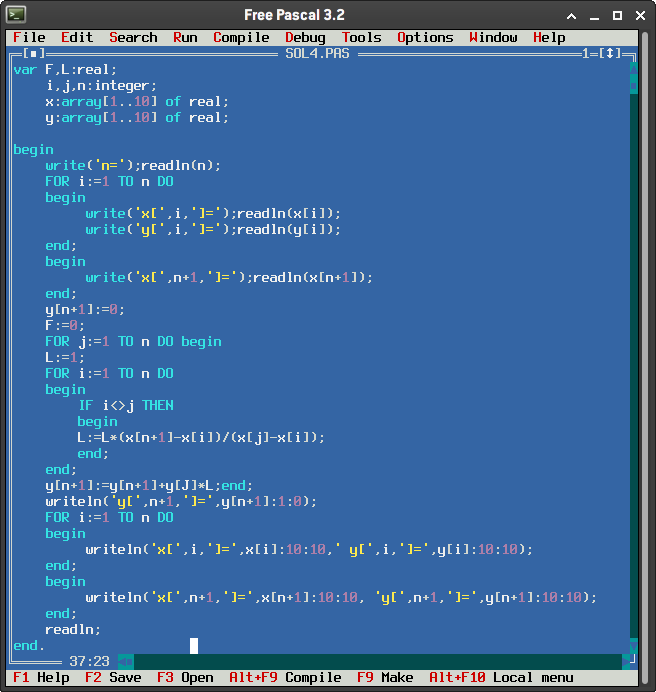
If some of you remember the MS-DOS with its black screen and blinking cursor, then that’s what I tried to write code for in 2005. By the way, I had no idea what I was doing, and because of the teachers we had, this whole experience kind of killed my desire to learn to program. Now, I’m 32 and decided to give coding another chance (since I feel more and more that it’s an important skill to have nowadays).
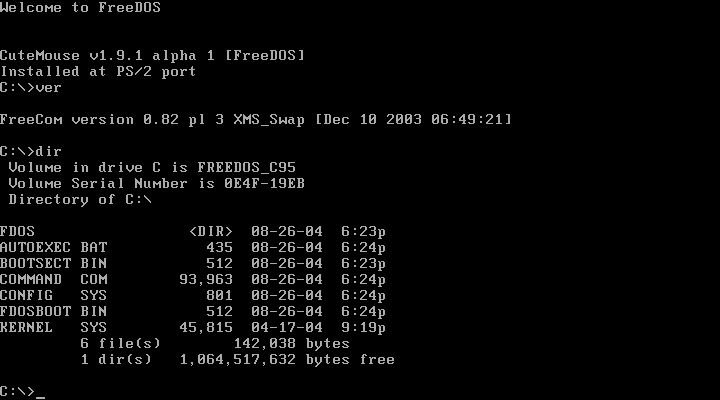
But without further ado, let’s start the notes for JavaScript since it’s the cockroach of programming languages (it lives forever).
Intro to JavaScript
I honestly hated the term JavaScript because during the times of the dial-up modems it was a very annoying thing and associated for me with pop-up windows. Now it became this modern coding language that everyone uses. It survived a lot while many other programming languages have perished or are perishing.
Let’s start with some basic terms.
Variables
A variable is a named space in memory.
Pretty much, when we assign a variable to the RAM, we are giving that piece of memory a “friendly name”. We can assign data to a variable, so we can access it later.
let name = "alex";
var age = 32;
We must create descriptive names for our variables, so we will be able to understand them later. Other coders might read the code, and we, as humans, tend to forget a lot of things. So, in order to avoid confusion for ourselves as well, we need to create the variables we will understand in the future.
Variable names are case-sensitive, they can start with a letter (or $ and _), and can contain numbers, letters, and $.
Reserved keywords cannot be variables.
Declaration of a Variable
A variable can be declared with either “let” or “var” keywords.
The differences between the two:
- Let: The values are only limited to the code block (what’s between the curly brackets) where it’s been declared. If a function will have more than one code block, the variable will be different in each of these blocks.
- Var: This variable will be considered the same within the function it’s been declared in no matter how many code blocks there are.
So, the difference between these two declaration words is scoping.
If a variable hasn’t been declared, it does not exist!
If a variable has been declared, you can assign or reassign values to it.
let randomNumber = 2; // Assigning the value during the declaration
randomNumber = 3; // Re-assigning the value
let anotherNumber; // Simply declaring the value
anotherNumber = 100; // Assigning the value to a previously declared variable
let number1, number2, number3; // Declaring multiple variables at once
Casing
There are two most common casings in JavaScript.
Camel Casing: the best practice when naming variables in JavaScript. The first letter is always lowercase. Imagine it like a camel 🐫 where each capital letter is a hump.
let camelCasing; // an example of Camel Casing.
Pascal Casing: not used in JavaScript that much, but it’s used in other languages. The first letter is capitalized.
let PascalCasing; // an example of Pascal Casing
Data Types
While learning intro to JavaScript, we have to mention data types. There is a multitude of data types across the programming languages. However, the most common types used in JS are:
- Number
- String
- Boolean
Numbers
Some programming languages (I’m looking at you, Pascal) have multiple variations for the numbers. However, in JavaScript, we have just one “number” data type. It includes integers and floats.
Note: In JavaScript, a float is accurate only to 7 decimal places.
What will the console.log() show?
let numberOne = 2;
let numberTwo = 3;
let sum = numberOne + numberTwo;
console.log(sum);
Strings
A string is a data type that represents multiple characters strung together.
We write values for the strings in quotes. Always.
let name = "Alex"; // This is a string
We can combine the strings using the “+” sign. It is called string concatenation.
let firstName = "Alex";
let lastName = "Pyslarash";
let fullName = firstName + " " + lastName;
console.log(fullName);
Booleans
A boolean is a data type that is only true or false.
let alexIsAwesome = true;
console.log(alexIsAwesome);
Dynamically-Typed Language
JavaScript is a dynamically-typed language.
It means that data types are not determined until the run time. And this is why we declare the variables with “let” or “var” regardless of the type.
Some other languages (like C#) are statically-typed. It means that data types are determined at compile time.
Pascal is a statically-typed language. We used to declare the type of the variable at the beginning of the code constantly. And there were many sub-types of the data types as well.
Arithmetic Operators
Let’s do some math in this intro to JavaScript.
Here are some of the operators included in JavaScript:
Operator | Description |
---|---|
+ | Addition |
– | Subtraction |
* | Multiplication |
/ | Division |
% | Modulus* |
++ | Increment |
— | Decrement |
*what modulus does is it shows the remainder after you divide one number by another. For example, if you divide 5 by 2, the remainder will be 1. Because 2+2+1=5.
Order of Operations
- Parentheses
- Exponents
- Multiply/Divide
- Add/Subtract
- Left to Right
Assignment Operators
In some cases, we can shorten the formula to save time and code space. Here are some of the common ways to do it:
Operator | Example | Equivalent |
---|---|---|
= | x = y | x = y |
+= | x += y | x = x + y |
-= | x -= y | x = x – y |
*= | x *= y | x = x * y |
/= | x /= y | x = x / y |
%= | x %= y | x = x % y |
++ | x++ | x = x + 1 |
— | x– | x = x – 1 |
Comparison Operators
Operator | Description |
---|---|
=== | Equal value and equal type |
!== | Unequal value and unequal type |
== | Equal value |
!= | Unequal value |
> | Greater than |
< | Less than |
>= | Greater than or equal to |
<= | Less than or equal to |
Comparison operators are used to compare two values to each other.
A comparison expression will always result in a boolean.
Conclusion
Well, this was the intro to JavaScript. We talked about variables, data types, and arithmetic operators. If you have any questions, feel free to leave a comment below. I hope that these notes were quite helpful if you are just beginning to code.